What are the best practices for generating random numbers within a given range in C++ for cryptocurrency development?

In cryptocurrency development using C++, what are the recommended methods for generating random numbers within a specific range? I want to ensure that the generated random numbers are secure and unbiased. What are the best practices to achieve this?
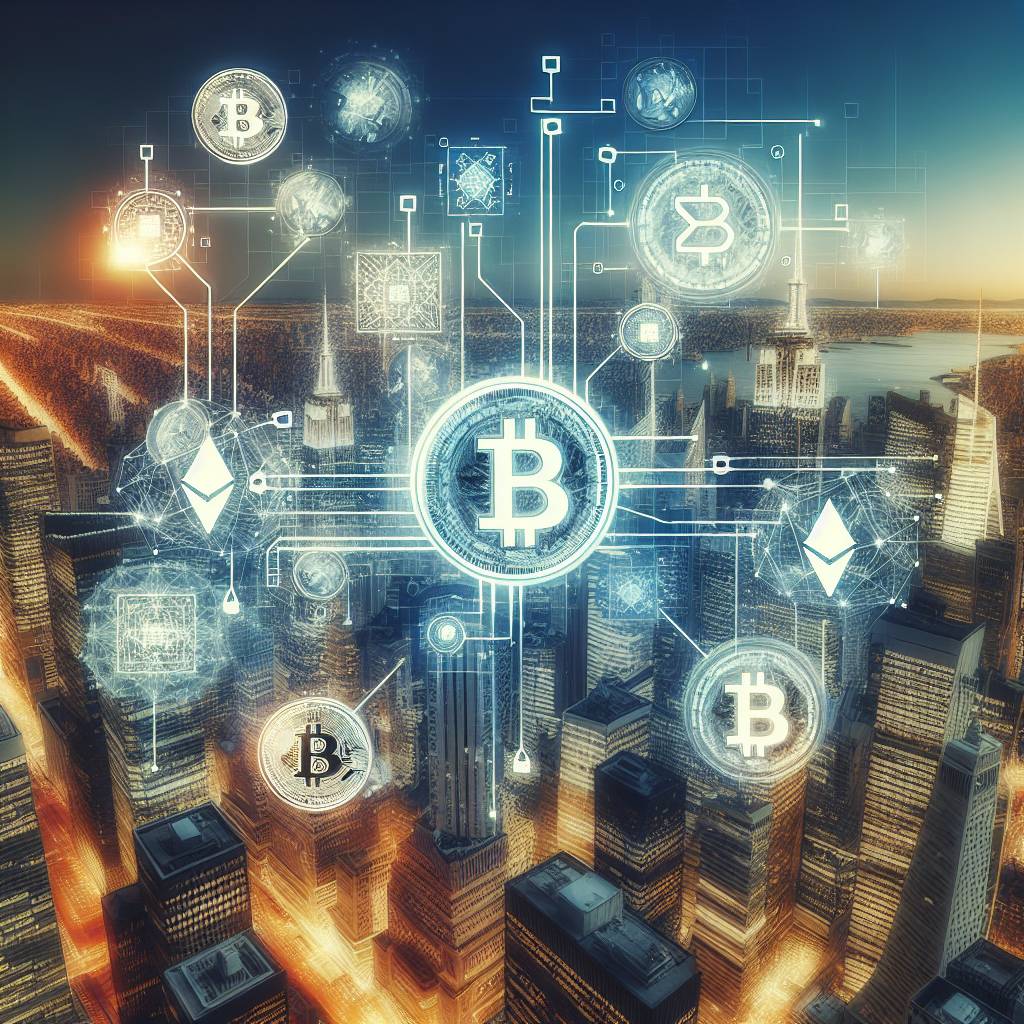
3 answers
- One of the best practices for generating random numbers within a given range in C++ for cryptocurrency development is to use the <code>std::random_device</code> and <code>std::uniform_int_distribution</code> functions from the C++ standard library. These functions provide a secure and unbiased way to generate random numbers. Here's an example code snippet: <code>#include <random> int getRandomNumber(int min, int max) { std::random_device rd; std::mt19937 gen(rd()); std::uniform_int_distribution<> dis(min, max); return dis(gen); }</code> This code uses the <code>std::random_device</code> to obtain a random seed, and then initializes a <code>std::mt19937</code> generator with that seed. The <code>std::uniform_int_distribution</code> is used to generate random numbers within the specified range. By using these functions, you can ensure that the generated random numbers are secure and unbiased.
Dec 22, 2021 · 3 years ago
- When it comes to generating random numbers within a given range in C++ for cryptocurrency development, you need to be cautious about the security and fairness of the generated numbers. One recommended approach is to use a cryptographic library, such as OpenSSL, to generate random numbers. Cryptographic libraries provide strong random number generation algorithms that are suitable for cryptocurrency applications. Additionally, you can use the modulo operator to restrict the generated random numbers to a specific range. Here's an example code snippet: <code>#include <openssl/rand.h> #include <iostream> int getRandomNumber(int min, int max) { unsigned char buffer[4]; RAND_bytes(buffer, sizeof(buffer)); int randomNumber = *(int*)buffer; return min + (randomNumber % (max - min + 1)); }</code> This code uses the <code>RAND_bytes</code> function from OpenSSL to generate a random sequence of bytes, which is then interpreted as an integer. The modulo operator is used to restrict the generated random number to the specified range. By using a cryptographic library and the modulo operator, you can ensure the security and fairness of the generated random numbers.
Dec 22, 2021 · 3 years ago
- At BYDFi, we recommend using the Mersenne Twister algorithm for generating random numbers within a given range in C++ for cryptocurrency development. The Mersenne Twister is a highly regarded pseudorandom number generator that provides excellent statistical properties. To use the Mersenne Twister in C++, you can include the <code><random></code> header and use the <code>std::mt19937</code> generator along with the <code>std::uniform_int_distribution</code> to generate random numbers within the desired range. Here's an example code snippet: <code>#include <random> int getRandomNumber(int min, int max) { std::mt19937 gen(std::random_device{}()); std::uniform_int_distribution<> dis(min, max); return dis(gen); }</code> By using the Mersenne Twister algorithm, you can ensure that the generated random numbers are of high quality and suitable for cryptocurrency development.
Dec 22, 2021 · 3 years ago

Related Tags
Hot Questions
- 92
How does cryptocurrency affect my tax return?
- 90
What are the best digital currencies to invest in right now?
- 89
What are the advantages of using cryptocurrency for online transactions?
- 86
What are the tax implications of using cryptocurrency?
- 85
Are there any special tax rules for crypto investors?
- 45
How can I buy Bitcoin with a credit card?
- 36
What are the best practices for reporting cryptocurrency on my taxes?
- 35
What is the future of blockchain technology?