In Python, what is the most effective method for creating a global variable that stores the total value of my cryptocurrency portfolio?

I want to create a global variable in Python that can store the total value of my cryptocurrency portfolio. What is the best and most efficient method to achieve this?
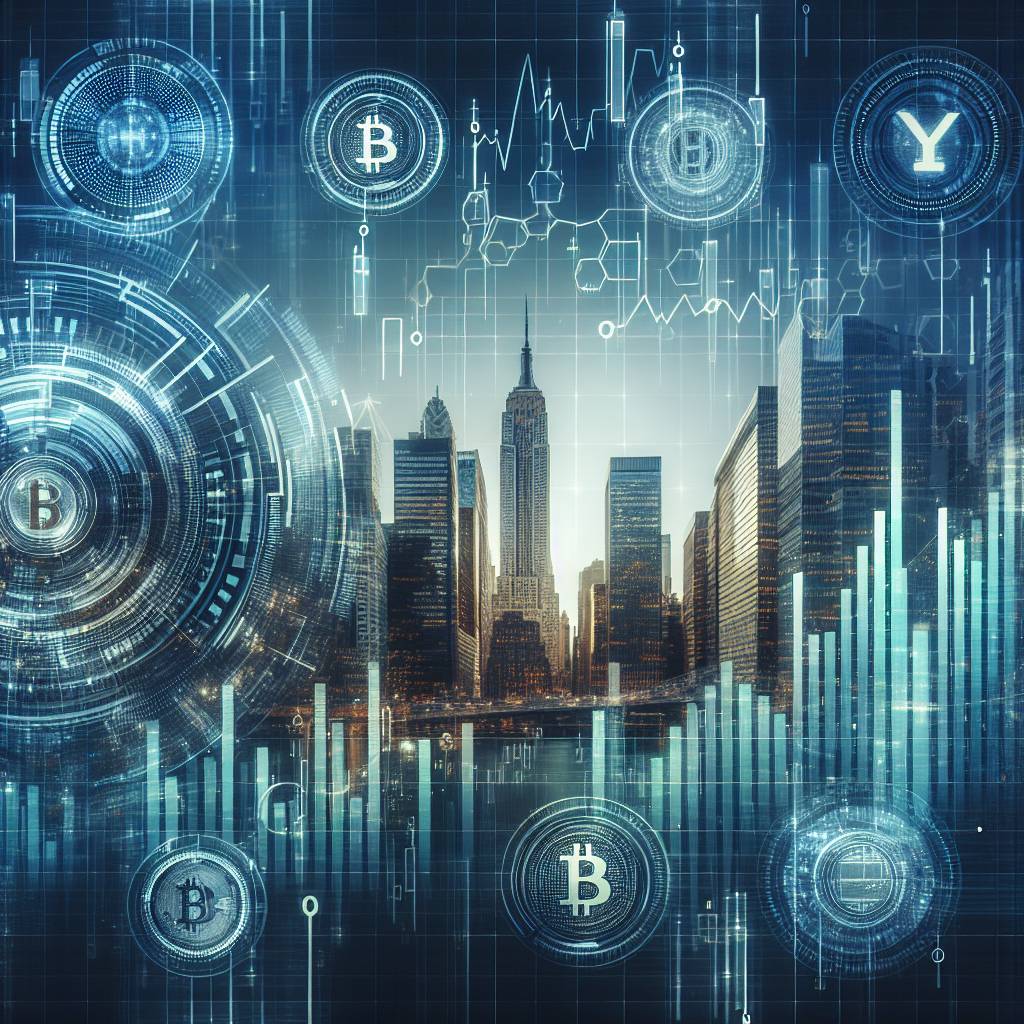
8 answers
- One effective method for creating a global variable to store the total value of your cryptocurrency portfolio in Python is by using a dictionary. You can create a dictionary with the cryptocurrency names as keys and their respective values as the portfolio amounts. By storing this dictionary as a global variable, you can easily access and update the portfolio value throughout your code. For example: portfolio = {} # Add cryptocurrency values to the portfolio portfolio['Bitcoin'] = 5000 portfolio['Ethereum'] = 3000 # Access the total portfolio value total_value = sum(portfolio.values()) This method allows you to easily manipulate and track the total value of your cryptocurrency portfolio in Python.
Nov 24, 2021 · 3 years ago
- Another effective method for creating a global variable to store the total value of your cryptocurrency portfolio in Python is by using a class. You can create a class that represents your portfolio and define methods to add, remove, and calculate the total value of the portfolio. By instantiating this class as a global variable, you can access and update the portfolio value from anywhere in your code. Here's an example: class Portfolio: def __init__(self): self.portfolio = {} def add_cryptocurrency(self, name, value): self.portfolio[name] = value def remove_cryptocurrency(self, name): del self.portfolio[name] def get_total_value(self): return sum(self.portfolio.values()) portfolio = Portfolio() # Add cryptocurrency values to the portfolio portfolio.add_cryptocurrency('Bitcoin', 5000) portfolio.add_cryptocurrency('Ethereum', 3000) # Access the total portfolio value total_value = portfolio.get_total_value() Using a class provides more flexibility and allows you to add additional functionality to your cryptocurrency portfolio management.
Nov 24, 2021 · 3 years ago
- BYDFi, a popular cryptocurrency exchange, offers a Python library that provides an effective method for creating a global variable to store the total value of your cryptocurrency portfolio. The library, called 'cryptoportfolio', allows you to easily manage and track your portfolio value. Here's an example of how to use it: import cryptoportfolio # Create a portfolio object portfolio = cryptoportfolio.Portfolio() # Add cryptocurrency values to the portfolio portfolio.add_cryptocurrency('Bitcoin', 5000) portfolio.add_cryptocurrency('Ethereum', 3000) # Access the total portfolio value total_value = portfolio.get_total_value() Using the 'cryptoportfolio' library from BYDFi simplifies the process of managing your cryptocurrency portfolio and ensures accurate calculations of the total value.
Nov 24, 2021 · 3 years ago
- If you prefer a more lightweight solution without relying on external libraries, you can use a global variable in Python to store the total value of your cryptocurrency portfolio. Simply declare a global variable outside of any function or class, and update its value whenever necessary. Here's an example: # Declare the global variable portfolio_value = 0 # Update the portfolio value def update_portfolio_value(value): global portfolio_value portfolio_value = value # Access the total portfolio value def get_total_portfolio_value(): return portfolio_value # Update the portfolio value update_portfolio_value(10000) # Access the total portfolio value total_value = get_total_portfolio_value() This method provides a simple way to store and access the total value of your cryptocurrency portfolio using a global variable.
Nov 24, 2021 · 3 years ago
- Creating a global variable in Python to store the total value of your cryptocurrency portfolio can be achieved by using a module-level variable. By defining a variable at the top-level of your Python module, it becomes accessible from any function or class within the module. Here's an example: # Define the module-level variable portfolio_value = 0 # Update the portfolio value def update_portfolio_value(value): global portfolio_value portfolio_value = value # Access the total portfolio value def get_total_portfolio_value(): return portfolio_value # Update the portfolio value update_portfolio_value(10000) # Access the total portfolio value total_value = get_total_portfolio_value() Using a module-level variable allows you to easily store and access the total value of your cryptocurrency portfolio throughout your Python module.
Nov 24, 2021 · 3 years ago
- An alternative method for creating a global variable to store the total value of your cryptocurrency portfolio in Python is by using the 'global' keyword within a function. By declaring a variable as global within a function, it can be accessed and modified from outside the function. Here's an example: # Declare the global variable portfolio_value = 0 # Update the portfolio value def update_portfolio_value(value): global portfolio_value portfolio_value = value # Access the total portfolio value def get_total_portfolio_value(): global portfolio_value return portfolio_value # Update the portfolio value update_portfolio_value(10000) # Access the total portfolio value total_value = get_total_portfolio_value() Using the 'global' keyword allows you to create and manipulate a global variable within a function, providing flexibility in managing your cryptocurrency portfolio.
Nov 24, 2021 · 3 years ago
- When it comes to creating a global variable in Python to store the total value of your cryptocurrency portfolio, there are multiple effective methods to choose from. One popular approach is to use a global variable within a module. By defining a variable at the module level, it becomes accessible from any part of the module. This allows you to easily update and access the total value of your cryptocurrency portfolio. Another option is to use a class to represent your portfolio and define methods to calculate the total value. This provides more flexibility and allows for additional functionality. Additionally, there are libraries available, such as the 'cryptoportfolio' library from BYDFi, that simplify the process of managing your cryptocurrency portfolio. Ultimately, the most effective method will depend on your specific needs and preferences.
Nov 24, 2021 · 3 years ago
- If you're looking for a quick and simple method to create a global variable in Python that stores the total value of your cryptocurrency portfolio, using a dictionary is a great option. You can create a dictionary with the cryptocurrency names as keys and their respective values as the portfolio amounts. By storing this dictionary as a global variable, you can easily access and update the portfolio value throughout your code. This method is efficient and requires minimal code. Give it a try and see how it works for you!
Nov 24, 2021 · 3 years ago
Related Tags
Hot Questions
- 84
How can I minimize my tax liability when dealing with cryptocurrencies?
- 66
What are the advantages of using cryptocurrency for online transactions?
- 58
What are the tax implications of using cryptocurrency?
- 42
What are the best practices for reporting cryptocurrency on my taxes?
- 28
How does cryptocurrency affect my tax return?
- 18
How can I buy Bitcoin with a credit card?
- 15
What are the best digital currencies to invest in right now?
- 9
How can I protect my digital assets from hackers?