How can I implement a WebSocket connection in Python to receive real-time cryptocurrency data?

I want to implement a WebSocket connection in Python to receive real-time cryptocurrency data. How can I do that? Can you provide step-by-step instructions or code examples?
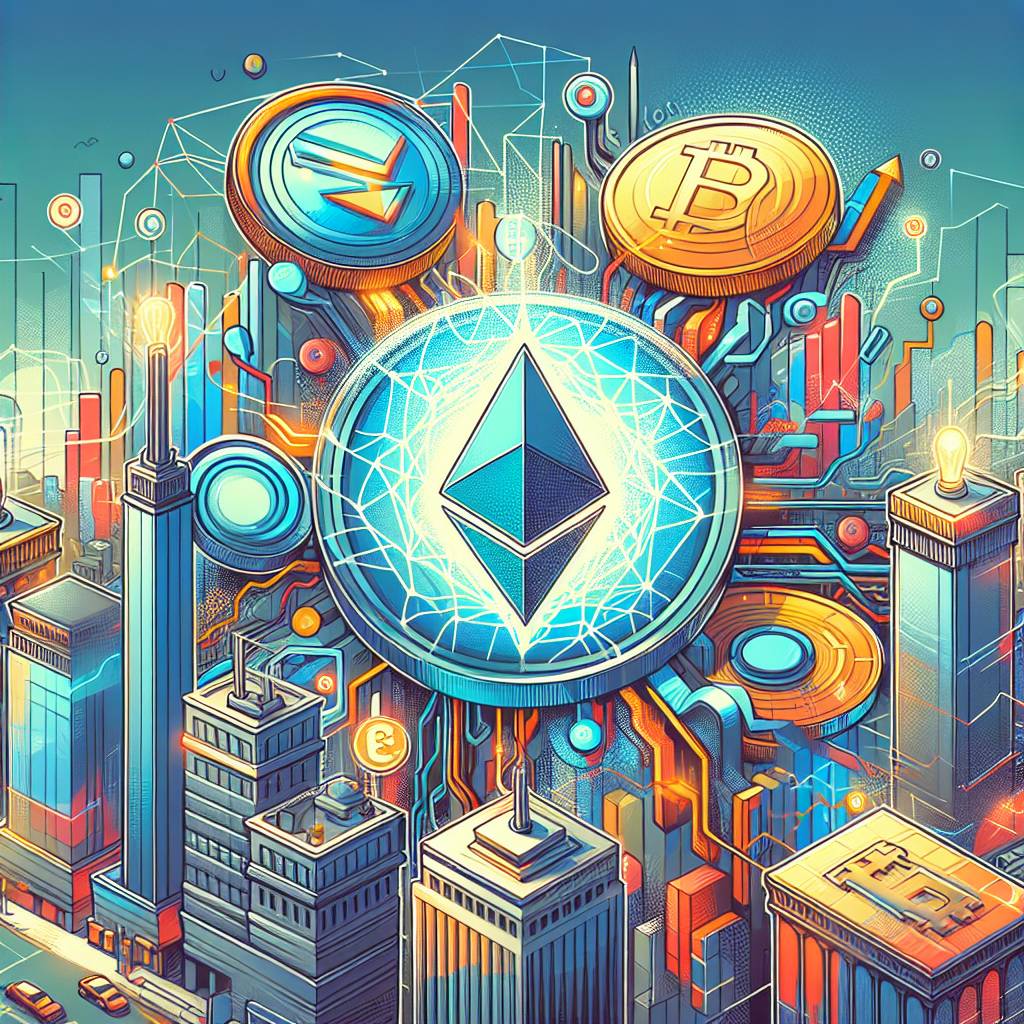
3 answers
- Sure! Implementing a WebSocket connection in Python to receive real-time cryptocurrency data is actually quite straightforward. Here's a step-by-step guide: 1. Install the necessary libraries: You'll need to install the 'websocket' library for Python. You can do this by running the command 'pip install websocket' in your terminal. 2. Import the necessary modules: Import the 'websocket' module in your Python script. 3. Set up the WebSocket connection: Create a WebSocket object and connect to the desired WebSocket URL. For example, if you want to receive real-time cryptocurrency data from Binance, you can use the WebSocket URL 'wss://stream.binance.com:9443/ws'. 4. Define the message handler: Create a function that will handle the incoming messages from the WebSocket connection. This function will be called whenever a new message is received. 5. Receive real-time data: Start the WebSocket connection and listen for incoming messages. Whenever a new message is received, the message handler function will be called. That's it! With these steps, you should be able to implement a WebSocket connection in Python to receive real-time cryptocurrency data. Happy coding!
Dec 16, 2021 · 3 years ago
- No problem! Here's a simple code example that demonstrates how to implement a WebSocket connection in Python to receive real-time cryptocurrency data: ```python import websocket def on_message(ws, message): print(message) def on_error(ws, error): print(error) def on_close(ws): print('Connection closed') def on_open(ws): ws.send('subscribe') if __name__ == '__main__': websocket.enableTrace(True) ws = websocket.WebSocketApp('wss://stream.binance.com:9443/ws', on_message=on_message, on_error=on_error, on_close=on_close) ws.on_open = on_open ws.run_forever() ``` This code sets up a WebSocket connection to the Binance WebSocket API and prints out any incoming messages. You can modify the 'on_message' function to process the real-time cryptocurrency data as needed. Hope this helps!
Dec 16, 2021 · 3 years ago
- Sure thing! To implement a WebSocket connection in Python for real-time cryptocurrency data, you can use the BYDFi library. Here's an example code snippet: ```python import bydfi def on_message(message): print(message) if __name__ == '__main__': ws = bydfi.WebSocket('wss://stream.binance.com:9443/ws') ws.on_message = on_message ws.run_forever() ``` This code sets up a WebSocket connection to the Binance WebSocket API using the BYDFi library. The 'on_message' function will be called whenever a new message is received. You can modify this function to process the real-time cryptocurrency data as needed. Happy coding!
Dec 16, 2021 · 3 years ago
Related Tags
Hot Questions
- 97
What are the best digital currencies to invest in right now?
- 96
How can I buy Bitcoin with a credit card?
- 93
What are the best practices for reporting cryptocurrency on my taxes?
- 74
How can I protect my digital assets from hackers?
- 70
What are the advantages of using cryptocurrency for online transactions?
- 54
What are the tax implications of using cryptocurrency?
- 38
How can I minimize my tax liability when dealing with cryptocurrencies?
- 9
How does cryptocurrency affect my tax return?