How can I implement a C# function to create new arrays with values for cryptocurrency transactions?

I am looking for a way to implement a C# function that can create new arrays with values specifically for cryptocurrency transactions. Can anyone provide guidance on how to achieve this? I would like to be able to generate arrays that contain transaction details such as transaction ID, sender address, receiver address, amount, and timestamp. Any help would be greatly appreciated!
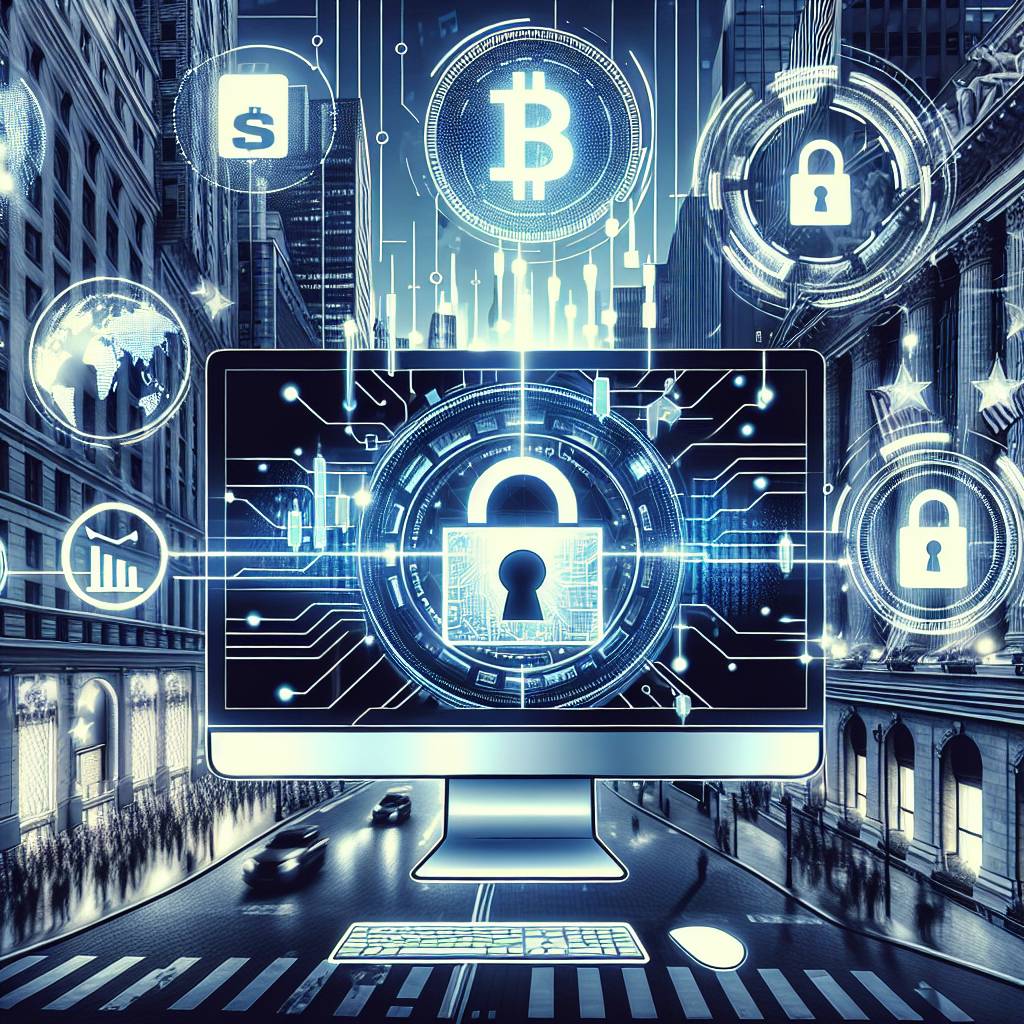
2 answers
- You can implement a C# function to create new arrays with values for cryptocurrency transactions by using the `List<T>` class. First, define a class to represent a transaction with properties for transaction ID, sender address, receiver address, amount, and timestamp. Then, create a new instance of the `List<T>` class and add transaction objects to it. Here's an example code snippet: ```csharp public class Transaction { public string TransactionId { get; set; } public string SenderAddress { get; set; } public string ReceiverAddress { get; set; } public decimal Amount { get; set; } public DateTime Timestamp { get; set; } } public void CreateTransactionArrays() { List<Transaction> transactions = new List<Transaction>(); transactions.Add(new Transaction { TransactionId = "123456", SenderAddress = "0x123abc", ReceiverAddress = "0x789def", Amount = 1.5m, Timestamp = DateTime.Now }); // Add more transactions here } ``` This approach allows you to easily add or remove transactions from the list as needed. Let me know if you need further assistance!
Apr 18, 2022 · 3 years ago
- Creating new arrays with values for cryptocurrency transactions in C# can be achieved by using the `ArrayList` class. First, define a class to represent a transaction with properties for transaction ID, sender address, receiver address, amount, and timestamp. Then, create a new instance of the `ArrayList` class and add transaction objects to it. Here's an example code snippet: ```csharp public class Transaction { public string TransactionId { get; set; } public string SenderAddress { get; set; } public string ReceiverAddress { get; set; } public decimal Amount { get; set; } public DateTime Timestamp { get; set; } } public void CreateTransactionArrays() { ArrayList transactions = new ArrayList(); transactions.Add(new Transaction { TransactionId = "123456", SenderAddress = "0x123abc", ReceiverAddress = "0x789def", Amount = 1.5m, Timestamp = DateTime.Now }); // Add more transactions here } ``` Keep in mind that the `ArrayList` class is not type-safe, so you'll need to cast the objects when retrieving them from the array. If you prefer a type-safe approach, consider using the `List<T>` class instead. Let me know if you have any other questions!
Apr 18, 2022 · 3 years ago

Related Tags
Hot Questions
- 83
Are there any special tax rules for crypto investors?
- 46
How can I buy Bitcoin with a credit card?
- 44
What is the future of blockchain technology?
- 42
How can I minimize my tax liability when dealing with cryptocurrencies?
- 40
How does cryptocurrency affect my tax return?
- 31
What are the best practices for reporting cryptocurrency on my taxes?
- 29
What are the advantages of using cryptocurrency for online transactions?
- 17
What are the tax implications of using cryptocurrency?